|
|
Title | Create menu items at run time with images, shortcut keys, and event handlers in Visual Basic .NET |
Description | This example shows how to create menu items at run time with images, shortcut keys, and event handlers in Visual Basic .NET. |
Keywords | menus, runtime menus, run time menus, create menus, create menus at runtime, create menus at run time, Visual Basic .NET, VB.NET |
Categories | Controls |
|
|
This example uses the following code to add new menu items to the Tools menu when the program starts.
|
|
' Create some tool menu items.
Private Sub Form1_Load(ByVal sender As System.Object, ByVal _
e As System.EventArgs) Handles MyBase.Load
' Tool 1 displays a string.
Dim tool1 As New ToolStripMenuItem("Tool 1")
tool1.Name = "mnuToolsTool1"
tool1.ShortcutKeys = (Keys.D1 Or Keys.Control) ' Ctrl+1
AddHandler tool1.Click, AddressOf mnuTool1_Click
mnuTools.DropDownItems.Add(tool1)
' Tool 2 displays a string and image.
Dim tool2 As New ToolStripMenuItem( _
"Tool 2", My.Resources.happy)
tool2.Name = "mnuToolsTool2"
tool2.ShortcutKeys = (Keys.D2 Or Keys.Control) ' Ctrl+2
AddHandler tool2.Click, AddressOf mnuTool2_Click
mnuTools.DropDownItems.Add(tool2)
End Sub
|
|
The code first creates a ToolStripMenuItem, passing the constructor the string it should display. It then:
- Sets the item's name
- Sets the item's ShortcutKeys property to the 1 key plus the Control key so it is activated when the user presses Ctrl+1
- Adds the mnuTool1_Click event handler to the item's Click event handler
The code then adds the new item to the mnuTools top-level menu item created at design time.
The program repeats these steps for a second menu item, this time passing the ToolStripMenuItem constructor a string and image so the menu item displays both text and a picture.
The following code shows the mnuTool1_Click event handler. The mnuTool2_Click event handler is similar.
|
|
' Execute tool 1.
Private Sub mnuTool1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs)
MessageBox.Show("Tool 1")
End Sub
|
|
|
|
|
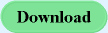 |
|
|
|