|
|
Title | Draw a non-triangular Sierpinski gasket (fractal) in VB.NET |
Description | This example shows how to draw a non-triangular Sierpinski gasket (fractal) in in VB.NET. |
Keywords | Sierpinski gasket, fractal, random, VB.NET |
Categories | Graphics |
|
|
When the user left-clicks, the program saves the point to use as a corner. When the user right-clicks, the program calls subroutine PlotPoints to draw the fractal.
PlotPoints picks a random corner to use as a starting point. It the repeatedly picks a new random corner and moves halfway from the current point to this corner, and plots the resulting point. When this process repeats enough times, the program draws a Sierpinski gasket.
If you cover and expose the form, the program draws the same Sierpinski gasket.
|
|
Private m_Running As Boolean
Private m_NumPoints As Integer
Private m_CornerX() As Integer
Private m_CornerY() As Integer
Private Const RADIUS As Integer = 5
Private Sub Form1_Load(ByVal sender As System.Object, ByVal _
e As System.EventArgs) Handles MyBase.Load
Randomize()
End Sub
Private Sub Form1_MouseDown(ByVal sender As Object, ByVal e _
As System.Windows.Forms.MouseEventArgs) Handles _
MyBase.MouseDown
If m_Running Then
' Stop running.
m_Running = False
m_NumPoints = 0
Else
' Left or right button?
If e.Button = MouseButtons.Left Then
' If this is the first point,
' erase the previous picture.
Dim gr As Graphics = Me.CreateGraphics()
If m_NumPoints = 0 Then gr.Clear(Me.BackColor)
' Save the point.
m_NumPoints = m_NumPoints + 1
ReDim Preserve m_CornerX(m_NumPoints - 1)
ReDim Preserve m_CornerY(m_NumPoints - 1)
m_CornerX(m_NumPoints - 1) = e.X
m_CornerY(m_NumPoints - 1) = e.Y
gr.DrawEllipse(Pens.Blue, e.X - RADIUS \ 2, e.Y _
- RADIUS \ 2, RADIUS, RADIUS)
Else
' See if we have enough points.
If m_NumPoints < 2 Then
' We need more points.
MsgBox("You must left-click at least two " & _
"points before right-clicking.", _
vbExclamation, "Need More Points")
Else
' Start.
m_Running = True
PlotPoints()
End If
End If
End If
End Sub
Private Sub PlotPoints()
Dim i As Integer
Dim last_x As Single
Dim last_y As Single
' Pick a starting point.
i = CInt(Int(Rnd() * m_NumPoints))
last_x = m_CornerX(i)
last_y = m_CornerY(i)
' Plot points until the user clicks again.
Dim gr As Graphics = Me.CreateGraphics()
Do While m_Running
' Pick the next corner.
i = CInt(Int(Rnd() * m_NumPoints))
' Move halfway from the current point
' to the new corner.
last_x = (last_x + m_CornerX(i)) / 2
last_y = (last_y + m_CornerY(i)) / 2
gr.DrawLine(Pens.Red, last_x, last_y, last_x + 1, _
last_y + 1)
' Check for events.
Application.DoEvents()
Loop
End Sub
Private Sub Form1_Closing(ByVal sender As Object, ByVal e _
As System.ComponentModel.CancelEventArgs) Handles _
MyBase.Closing
End
End Sub
|
|
Experiment with different corner points. Try a triangle, rectangle (you may need to use code to position the points exactly), and pentagon. Try a rectangle with three extra points at the same spot in the middle.
For more information on drawing fractals and other graphics, see my book Visual Basic Graphics Programming.
|
|
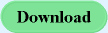 |
|
|
|