|
|
Title | Draw a Sierpinski gasket (fractal) |
Description | This example shows how to draw a Sierpinski gasket (fractal) in Visual Basic 6. |
Keywords | Sierpinski gasket, fractal, random |
Categories | Graphics |
|
|
The program defines three corner points and picks a random corner to use as a starting point. It the repeatedly picks a new random corner and moves halfway from the current point to this corner, and plots the resulting point. When this process repeats enough times, the program draws a Sierpinski gasket.
If you resize the program, it clears, defines new corner points, and starts over. If you cover and expose the form, the program draws the same Sierpinski gasket.
|
|
Private LastX As Single
Private LastY As Single
Private CornerX(0 To 2) As Single
Private CornerY(0 To 2) As Single
' Draw the Sierpinski gasket.
Private Sub Form_Load()
Dim i As Integer
Randomize
Me.Show
Do
' Pick the next corner.
i = Int(Rnd * 3)
' Move halfway from the current point
' to the new corner.
LastX = (LastX + CornerX(i)) / 2
LastY = (LastY + CornerY(i)) / 2
Me.PSet (LastX, LastY)
' Check for events.
DoEvents
Loop
End Sub
' Define the corner points.
Private Sub Form_Resize()
Const R As Single = 60
Dim i As Integer
' Clear.
Me.Cls
' Define the corners.
CornerX(0) = R + 10
CornerY(0) = Me.ScaleHeight - R - 10
CornerX(1) = Me.ScaleWidth / 2
CornerY(1) = R + 10
CornerX(2) = Me.ScaleWidth - R - 10
CornerY(2) = Me.ScaleHeight - R - 10
' Draw the corners.
For i = 0 To 2
Me.Circle (CornerX(i), CornerY(i)), R
Next i
' Pick a starting point.
i = Int(Rnd * 3)
LastX = CornerX(i)
LastY = CornerY(i)
End Sub
Private Sub Form_Unload(Cancel As Integer)
End
End Sub
|
|
For more information on drawing fractals and other graphics, see my book Visual Basic Graphics Programming.
|
|
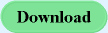 |
|
|
|