|
|
Title | Use animation to show how the recursive solution to the Tower of Hanoi problem works in Visual Basic 6 |
Description | This example shows how to use animation to show how the recursive solution to the Tower of Hanoi problem works in Visual Basic 6. |
Keywords | algorithms, recursion, Tower of Hanoi, games, puzzles, Visual Basic 6, VB 6 |
Categories | Algorithms, Multimedia |
|
|
This example is similar to the example Recursively solve the Tower of Hanoi problem in Visual Basic 6 except it uses the following AnimateMovement method to show how disks move from one peg to another.
|
|
' Move the moving disk to this location.
Private Sub AnimateMovement(ByVal end_x As Integer, ByVal _
end_y As Integer)
Const twips_per_second As Integer = 6000
Dim start_x As Integer
Dim start_y As Integer
Dim dx As Single
Dim dy As Single
Dim dist As Single
Dim seconds As Single
Dim start_time As Single
Dim elapsed As Single
start_x = MovingDiskRect.X
start_y = MovingDiskRect.Y
dx = end_x - MovingDiskRect.X
dy = end_y - MovingDiskRect.Y
dist = CSng(Sqr(dx * dx + dy * dy))
' Calculate distance moved per second.
dx = twips_per_second * dx / dist
dy = twips_per_second * dy / dist
' See how long the total move will take.
seconds = dist / twips_per_second
start_time = Timer
' Start moving.
Do
' Redraw.
Me.Refresh
' Wait a little while.
Dim stop_time As Single
stop_time = Timer + 0.01
Do While Timer < stop_time
DoEvents
Loop
' See how much time has passed.
elapsed = Timer - start_time
If (elapsed > seconds) Then Exit Do
' Update the rectangle's position.
MovingDiskRect.X = CInt(start_x + elapsed * dx)
MovingDiskRect.Y = CInt(start_y + elapsed * dy)
Loop
MovingDiskRect.X = end_x
MovingDiskRect.Y = end_y
End Sub
|
|
The code calculates the number of pixels per second it should add to the moving rectangle's X and Y coordinates. It then enters a loop where it adds the needed number of pixels, depending on how much time has elapsed.
Download the example for additional details.
|
|
|
|
|
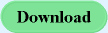 |
|
|
|