|
|
Title | Determine where two circles intersect in Visual Basic 6 |
Description | This example shows how to determine where two circles intersect in Visual Basic 6. |
Keywords | circles,intersect,intersect two circles,intersections,find circle intersections, VB 6, Visual Basic 6 |
Categories | Graphics, Algorithms |
|
|
If you don't like math, skip to the code below.
Consider the figure on the right showing two circles with radii r0 and r1. The points p0, p1, p2, and p3 have coordinates (x0, y0) and so forth.
Let d = the distance between the circles' centers so . Solving for a gives .Now there are three cases:
- If d > r0 + r1: The circles are too far apart to intersect.
- If d < |r0 - r1|: One circle is inside the other so there is no intersection.
- If d = 0 and r0 = r1: The circles are the same.
- If d = r0 + r1: The circles touch at a single point.
- Otherwise: The circles touch at two points.
The Pythagorean theorem gives:

So:

Substituting and multiplying this out gives:

The -b2 terms on each side cancel out. You can then solve for b to get:

Similarly:

All of these values are known so you can solve for a and b. All that remains is using those distances to find the points p3.
If a line points in direction <dx, dy>, then two perpendicular lines point in the directions <dy, -dx> and <-dy, dx>. Scaling the result gives the following coordinates for the points p3:
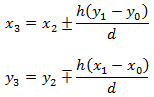
Be careful to notice the ± and ∓ symbols.
Click and drag to create two circles on the example program. The following code shows the FindCircleCircleIntersections method that the program uses to find the intersections.
|
|
' Find the points where the two circles intersect.
Private Function FindCircleCircleIntersections( _
ByVal cx0 As Single, ByVal cy0 As Single, ByVal radius0 _
As Single, _
ByVal cx1 As Single, ByVal cy1 As Single, ByVal radius1 _
As Single, _
ByRef intersectionx1 As Single, ByRef intersectiony1 As _
Single, _
ByRef intersectionx2 As Single, ByRef intersectiony2 As _
Single) As Integer
Dim dx, dy As Single
Dim dist, a, h, cx2, cy2 As Double
' Find the distance between the centers.
dx = cx0 - cx1
dy = cy0 - cy1
dist = Sqr(dx * dx + dy * dy)
' See how many solutions there are.
If (dist > radius0 + radius1) Then
' No solutions, the circles are too far apart.
intersectionx1 = NAN
intersectiony1 = NAN
intersectionx2 = NAN
intersectiony2 = NAN
FindCircleCircleIntersections = 0
Exit Function
ElseIf (dist < Math.Abs(radius0 - radius1)) Then
' No solutions, one circle contains the other.
intersectionx1 = NAN
intersectiony1 = NAN
intersectionx2 = NAN
intersectiony2 = NAN
FindCircleCircleIntersections = 0
ElseIf ((dist = 0) And (radius0 = radius1)) Then
' No solutions, the circles coincide.
intersectionx1 = NAN
intersectiony1 = NAN
intersectionx2 = NAN
intersectiony2 = NAN
FindCircleCircleIntersections = 0
Else
' Find a and h.
a = (radius0 * radius0 - _
radius1 * radius1 + dist * dist) / (2 * dist)
h = Sqr(radius0 * radius0 - a * a)
' Find P2.
cx2 = cx0 + a * (cx1 - cx0) / dist
cy2 = cy0 + a * (cy1 - cy0) / dist
' Get the points P3.
intersectionx1 = CSng(cx2 + h * (cy1 - cy0) / dist)
intersectiony1 = CSng(cy2 - h * (cx1 - cx0) / dist)
intersectionx2 = CSng(cx2 - h * (cy1 - cy0) / dist)
intersectiony2 = CSng(cy2 + h * (cx1 - cx0) / dist)
' See if we have 1 or 2 solutions.
If (dist = radius0 + radius1) Then
FindCircleCircleIntersections = 1
Else
FindCircleCircleIntersections = 2
End If
Exit Function
End If
End Function
|
|
|
|
|
|