|
|
Title | Use a Complex class to draw the Mandelbrot set easily in Visual Basic 6 |
Description | This example shows how to use a Complex class to draw the Mandelbrot set in Visual Basic 6. |
Keywords | Mandelbrot set, fractal, Visual Basic 6, VB 6, Visual Basic |
Categories | Graphics, Graphics, Algorithms |
|
|
You can draw a Mandelbrot set by iterating the equation:
Zn = Zn-12 + C
Where Zn and C are complex numbers. For a point (X, Y) in a particular area of the set, the program sets Z0 = 0 and C = X + Y * i. The program iterates this equation until the magnitude of Zn is at least 2 or the program performs a maximum number of iterations.
This example uses a Complex class to manage the complex numbers easily and intuitively. The following code shows the program's main loop.
|
|
Set Z = New Complex
Z.Initialize Z0.Real, Z0.Imaginary
Set C = New Complex
C.Initialize ReaC, ImaC
clr = 1
Do While clr < MaxIterations And Z.MagnitudeSquared() < _
MAX_MAG_SQUARED
' Calculate Z(clr).
Set Z = Z.Times(Z).Plus(C)
clr = clr + 1
Loop
' Set the pixel's value.
picCanvas.PSet (X, Y), QBColor(clr Mod 16)
|
|
The rest of the main program's code (and there's a lot of it) deals with resizing the form, letting you use the mouse to select an area for zooming, and so forth.
The following code shows the most important parts of the Complex class.
|
|
Public Real As Double
Public Imaginary As Double
Public Sub Initialize(ByVal new_Real As Double, ByVal _
new_Imaginary As Double)
Real = new_Real
Imaginary = new_Imaginary
End Sub
Public Function MagnitudeSquared() As Double
MagnitudeSquared = Real * Real + Imaginary * Imaginary
End Function
Public Function Magnitude() As Double
Magnitude = Sqr(Real * Real + Imaginary + Imaginary)
End Function
Public Function Times(ByVal c2 As Complex) As Complex
Dim result As Complex
Set result = New Complex
result.Real = Me.Real * c2.Real - Me.Imaginary * _
c2.Imaginary
result.Imaginary = Me.Real * c2.Imaginary + Me.Imaginary _
* c2.Real
Set Times = result
End Function
Public Function Plus(ByVal c2 As Complex) As Complex
Dim result As Complex
Set result = New Complex
result.Real = Me.Real + c2.Real
result.Imaginary = Me.Imaginary + c2.Imaginary
Set Plus = result
End Function
|
|
See the code for details.
Compare this example to Use a Complex class to draw the Mandelbrot set easily in Visual Basic .NET. That example has a few extra features that I haven't gotten aroundd to adding to this example (such as letting you pick the colors the program uses), but something you should look at is their performances. The .NET version is much faster.
|
|
|
|
|
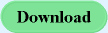 |
|
|
|