|
|
Title | Make extension methods to convert file sizes into strings giving sizes in KB, MB, etc. in Visual Basic .NET |
Description | This example shows how to make extension methods to convert file sizes into strings giving sizes in KB, MB, etc. in Visual Basic .NET. |
Keywords | ListBox, TextBox, set tabs, tabs, tab stops, set tab stops, Visual Basic .NET, VB.NET |
Categories | Software Engineering, Files and Directories |
|
|
This example defines two extension methods for converting a file size in bytes into a value ending with an appropriate size such as KB, MB, or GB.
The first method uses the StrFormatByteSize API function.
|
|
<System.Runtime.InteropServices.DllImport("shlwapi", _
CharSet:=CharSet.Auto)> _
Private Function StrFormatByteSize(ByVal fileSize As Long, _
ByVal buffer As StringBuilder, ByVal bufferSize As _
Integer) As Long
End Function
' Return a file size created by the StrFormatByteSize API
' function.
<Extension()> _
Public Function ToFileSizeApi(ByVal file_size As Long) As _
String
Dim sb As New StringBuilder(20)
StrFormatByteSize(file_size, sb, 20)
Return sb.ToString()
End Function
|
|
This gives the same result that Windows Explorer does for a file. Unfortunately the StrFormatByteSize API function uses long integers to store byte values so it can only handle up to 2,147,483,647 bytes or a bit less than 2 GB. Modern computers often work with values greater than 2 GB and in some cases more than 1 TB (1 terabyte = 1024 gigabytes).
The following extension method uses the Double data type to overcome this restriction.
|
|
' Return a string describing the value as a file size.
' For example, 1.23 MB.
<Extension()> _
Public Function ToFileSize(ByVal value As Double) As String
Dim suffixes As String() = {"bytes", "KB", "MB", "GB", _
"TB", "PB", "EB", "ZB", "YB"}
For i As Integer = 0 To suffixes.Length - 1
If (value <= (Math.Pow(1024, i + 1))) Then
Return ThreeNonZeroDigits(value / Math.Pow(1024, _
i)) & " " & suffixes(i)
End If
Next i
Return ThreeNonZeroDigits(value / Math.Pow(1024, _
suffixes.Length - 1)) & _
" " & suffixes(suffixes.Length - 1)
End Function
' Return the value formatted to include at most three
' non-zero digits and at most two digits after the
' decimal point. Examples:
' 1
' 123
' 12.3
' 1.23
' 0.12
Private Function ThreeNonZeroDigits(ByVal value As Double) _
As String
If (value >= 100) Then
' No digits after the decimal.
Return value.ToString("0,0")
ElseIf (value >= 10) Then
' One digit after the decimal.
Return value.ToString("0.0")
Else
' Two digits after the decimal.
Return value.ToString("0.00")
End If
End Function
|
|
|
|
|
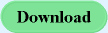 |
|
|
|