|
|
Title | Use EXIF information to orient an image properly in Visual Basic .NET |
Description | Use EXIF information to orient an image properly in Visual Basic .NET, |
Keywords | graphics, files, orient image, orient picture, image processing, EXIF, orientation, rotate image, example, example program, Windows Forms programming, Visual Basic .NET, VB.NET |
Categories | Algorithms, Graphics, Graphics, Files and Directories |
|
|
The example Read an image file's EXIF orientation data in Visual Basic .NET shows how to read EXIF orientation information from an image. Using that information, it's easy to orient the image so it appears right side up.
This example uses the following code to orient an image.
|
|
' Make an image to demonstrate orientations.
Public Function OrientationImage(ByVal orientation As _
ExifOrientations) As Image
Const size As Integer = 64
Dim bm As New Bitmap(size, size)
Using gr As Graphics = Graphics.FromImage(bm)
gr.Clear(Color.White)
gr.TextRenderingHint = _
System.Drawing.Text.TextRenderingHint.AntiAliasGridFit
' Orient the result.
Select Case (orientation)
Case ExifOrientations.TopLeft
Case ExifOrientations.TopRight
gr.ScaleTransform(-1, 1)
Case ExifOrientations.BottomRight
gr.RotateTransform(180)
Case ExifOrientations.BottomLeft
gr.ScaleTransform(1, -1)
Case ExifOrientations.LeftTop
gr.RotateTransform(90)
gr.ScaleTransform(-1, 1, MatrixOrder.Append)
Case ExifOrientations.RightTop
gr.RotateTransform(-90)
Case ExifOrientations.RightBottom
gr.RotateTransform(90)
gr.ScaleTransform(1, -1, MatrixOrder.Append)
Case ExifOrientations.LeftBottom
gr.RotateTransform(90)
End Select
' Translate the result to the center of the bitmap.
gr.TranslateTransform(size / 2, size / 2, _
MatrixOrder.Append)
Using string_format As New StringFormat()
string_format.LineAlignment = _
StringAlignment.Center
string_format.Alignment = StringAlignment.Center
Using the_font As New Font("Times New Roman", _
40, GraphicsUnit.Point)
If (orientation = ExifOrientations.Unknown) _
Then
gr.DrawString("?", the_font, _
Brushes.Black, 0, 0, string_format)
Else
gr.DrawString("F", the_font, _
Brushes.Black, 0, 0, string_format)
End If
End Using
End Using
End Using
Return bm
End Function
' Set the image's orientation.
Public Sub SetImageOrientation(ByVal img As Image, ByVal _
orientation As ExifOrientations)
' Get the index of the orientation property.
Dim orientation_index As Integer = _
Array.IndexOf(img.PropertyIdList, OrientationId)
' If there is no such property, do nothing.
If (orientation_index < 0) Then Return
' Set the orientation value.
Dim item As PropertyItem = _
img.GetPropertyItem(OrientationId)
item.Value(0) = orientation
img.SetPropertyItem(item)
End Sub
|
|
The OrientImage method calls the ImageOrientation method described in the earlier example to see how the image is oriented. It then calls the Image class's RotateFlip method to rotate and flip the image so it is right side up. It finishes by calling the SetImageOrientation method to make the Image object's orientation TopLeft, the normal right side up orientation.
The SetImageOrientation method find the index of the image's orientation ID property. If that ID is present, the method gets its PropertyItem, sets the item's Value to the new orientation, and then uses the Image object's SetPropertyItem method to set the new value.
|
|
|
|
|
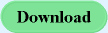 |
|
|
|