|
|
Title | Make thumbnails and a web page to display the images in a directory in Visual Basic .NET |
Description | This example shows how to make thumbnails and a web page to display the images in a directory in Visual Basic .NET. |
Keywords | files, internet, web, thumbnails, thumbnail images, web pages, HTML, Visual Basic .NET, VB.NET |
Categories | Files and Directories, Internet, Utilities |
|
|
This example searches a directory and builds a thumbnail image for each of the image files it finds there. It also builds a web page that displays the thumbnails and links to the full-scale images. It puts all of the files (original images, thumbnails, and web page) in the directory of your choice.
The following code shows the MakeWebPage method that does most of the work.
|
|
' Make the web page and thumbnails.
Private Sub MakeWebPage(ByVal input_dir As String, ByVal _
output_dir As String, ByVal url_prefix As String, ByVal _
web_page As String, ByVal thumb_width As Integer, ByVal _
thumb_height As Integer)
' Open the HTML file.
Dim html_filename As String = output_dir & web_page
Using html_file As New StreamWriter(html_filename)
' Make a list of the image files.
Dim files As List(Of String) = _
FindFiles(input_dir, _
"*.bmp;*.gif;*.jpg;*.png;*.tif", False)
' Process the files.
For Each image_filename As String In files
' Copy the file to the destination directory.
Dim image_fileinfo As New _
FileInfo(image_filename)
Dim dest_filename As String = output_dir & _
image_fileinfo.Name
File.Copy(image_filename, dest_filename, True)
' Get the image.
Using bm As New Bitmap(image_filename)
' Get the original size.
Dim src_rect As New Rectangle(0, 0, _
bm.Width, bm.Height)
' Shrink the image.
Dim scale As Double = Math.Min( _
CDbl(thumb_width) / bm.Width, _
CDbl(thumb_height) / bm.Height)
Dim shrunk_width As Integer = CInt(bm.Width _
* scale)
Dim shrunk_height As Integer = _
CInt(bm.Height * scale)
dim dest_rect as new Rectangle(0, 0, _
shrunk_width, shrunk_height)
Using thumbnail As New Bitmap(shrunk_width, _
shrunk_height)
' Copy the image at reduced scale.
Using gr As Graphics = _
Graphics.FromImage(thumbnail)
gr.DrawImage(bm, dest_rect, _
src_rect, GraphicsUnit.Pixel)
End Using
' Save the thumbnail image.
Dim thumb_filename As String = _
dest_filename.Substring(0, _
dest_filename.Length - _
image_fileinfo.Extension.Length) _
& _
"_thumb.png"
thumbnail.Save(thumb_filename, _
ImageFormat.Png)
' Add the thumbnail image to the HTML
' page.
Dim thumb_fileinfo As New _
FileInfo(thumb_filename)
html_file.WriteLine( _
"<a href=""" & url_prefix & _
image_fileinfo.Name & """>" & _
"<img src=""" & url_prefix & _
thumb_fileinfo.Name & """>" & _
"</a>")
End Using ' Using thumbnail As New
' Bitmap(shrunk_width, shrunk_height)
End Using ' Using bm As New
' Bitmap(image_filename)
Next image_filename
' Close the HTML file.
html_file.Close()
MessageBox.Show("Processed " & files.Count & "" & _
"images.")
End Using ' Using html_file As New
' StreamWriter(html_filename)
End Sub
|
|
The method starts by creating a StreamWriter so it can write the new web page. Next it calls the FindFiles method to get files in the target directory that have bmp, gif, jpg, png, or tif extensions. See the example Search for files that match multiple patterns in Visual Basic .NET for a description of the FindFiles method.
For each file it found, the code copies the file to the output directory. It then loads the file and calculates how big it should make the thumbnail to fit in the desired size without distorting it. The code makes a thumbnail bitmap of that size and copies the original image into it. The method saves the thumbnail in .png format and finally writes a line into the web page to display the thumbnail, linking it to the full-size image.
|
|
|
|
|
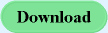 |
|
|
|