|
|
Title | Make SubArray extension methods that let you easily copy parts of one- and two-dimensional arrays in Visual Basic .NET |
Description | This example shows how to make SubArray extension methods that let you easily copy parts of one- and two-dimensional arrays in Visual Basic .NET. |
Keywords | copy two-dimensional array, copy 2-D array, two-dimensional arrays, 2-D arrays, example, example program, Windows Forms programming, Visual Basic .NET, VB.NET |
Categories | Software Engineering |
|
|
The Array class's Copy method can copy items from one array to another but it requires that you figure out how many items you want to copy. It will also copy parts of a two-dimensional array but it only copies contiguous pieces not rectangular chunks in the middle.
This example creates two SubArray extension methods that let you copy parts of a one- or two-dimensional array much as you can use a String's SubString method to copy parts of a String.
The following code shows the extension method that copies part of a one-dimensional array.
|
|
' Copy the indicated entries from an array into a new array.
<Extension()> _
Public Function SubArray(Of T)(ByVal values() As T, ByVal _
start_index As Integer, ByVal end_index As Integer) As _
T()
Dim num_items As Integer = end_index - start_index + 1
Dim result(0 To num_items - 1) As T
Array.Copy(values, start_index, result, 0, num_items)
Return result
End Function
|
|
This method calculates the number of items to copy and creates an array to hold that number of items. It uses Array.Copy to copy the desired items into the new array and returns the array.
The following code uses this extension method to copy items 1 through 3 of a one-dimensional array into a new array.
|
|
' Copy the array.
Dim new_values() As String = Values1d.SubArray(1, 3)
|
|
The following code shows the extension method that copies part of a two-dimensional array.
|
|
<Extension()> _
Public Function SubArray(Of T)(ByVal values(,) As T, ByVal _
row_min As Integer, ByVal row_max As Integer, ByVal _
col_min As Integer, ByVal col_max As Integer) As T(,)
Dim num_rows As Integer = row_max - row_min + 1
Dim num_cols As Integer = col_max - col_min + 1
Dim result(0 To num_rows - 1, 0 To num_cols - 1) As T
For row As Integer = 0 To num_rows - 1
For col As Integer = 0 To num_cols - 1
result(row, col) = values(row + row_min, col + _
col_min)
Next col
Next row
Return result
End Function
|
|
This method calculates the number of rows and columns it must copy and creates an array to hold the necessary number of items. It then loops through the desired items, copying them into the new array, and returns the new array.
The following code uses this extension method to copy the items in rows 2 - 3 and columns 1 - 3 into a new array.
|
|
' Copy the array.
Dim new_values(,) As String = Values2d.SubArray(2, 3, 1, 3)
|
|
|
|
|
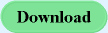 |
|
|
|